nikola-wan
Veteran Member
I saw posts on the Facebook BASIC Programming forum on various programs to calculate PI and decided to port one to Tektronix 4050 BASIC.
Tektronix 4050 BASIC was introduced in 1975 with their 4051 vector graphics microcomputer. The 4051 and the second generation 4052 and 4054 targeted commercial, scientific and military applications and only supported double-precision floating point numeric variables. Most of the other vintage computers BASIC included integer variable support, and the PI calculation programs I found made heavy use of integer variables.
Tektronix 4050 BASIC stores floating point variables in 8-bytes, so this significantly limits the number of numeric variables in a program. I have written programs that use character strings to emulate integer variables - but this is significantly slower due to the number of BASIC commands involved. PI calculations require significant size numeric arrays.
One of the shortest and fastest BASIC programs I found for calculating PI was the one written in BBC BASIC: https://rosettacode.org/wiki/Pi#BBC_BASIC
I was not familiar with that BASIC, but I had tried porting several other programs from that webpage - and new that Tektronix 4050 computers would be performance disadvantaged, so I decided to tackle porting the BBC BASIC program to Tektronix BASIC. Here is the BBC BASIC program listing:
This program is very short - and shows that BBC BASIC supports structured BASIC. I found a BBC BASIC for SDL 2.0 for windows here: https://www.bbcbasic.co.uk/bbcbasic.html
and found it easy to use and very quick - as it is compiled to run directly on windows.
I pasted the BBC Pi program into BBC BASIC window (I selected the Richard Russell SDLIDE version) and pressed the F9 function key and a console window popped up and began displaying the results of the Pi calculation and kept scrolling off the screen! I closed the console window and looked at the program and found one array B%() that was initialized to M%=(HIMEM)-END-1000). I tried replacing M% in the DIM B% statement with 50, pressed F9 and got an error message. I then added REM in front of the M%= calculation and added a M%=50, pressed f9 and the console window printed Pi to 11 decimal places! I then typed in the console window: PRINT HIMEM and got 192814076 (192MB)! At that point I experimented with changing the value of M% and developed an algorithm to predict the number of decimal places based on the value of M%.
I also began creating my port of the program in Tektronix 4050 BASIC using the latest experimental version of the Tek 405x (actually 4051) Web Browser Emulator:
https://forum.vcfed.org/index.php?threads/tek-405x-web-browser-emulator.62548/post-1357155
For developing my BASIC programs - I edit the mc6800.js file and replacing the 368 with 3.68 which increases the speed of the emulated Motorola 6800 CPU to about 100MHz for about 100x performance speed up of the 405x Emulator compared to the Tektronix 4051 and almost 10x speedup of the Emulator compared to my 4051 and 4052!
After hours of work - I have created this BASIC program that runs on the 405x Emulator in 32KB of RAM (and therefore should run on a 4051) and also tested it with my 4052. Since my program uses simpler BASIC calls to emulate the MOD, DIV, and print formatting of the BBC_PI program - I believe it will also be easier to port to other vintage computer BASIC dialects.
I have inserted blank lines in the listing for better readability. I have created several versions of this program: the listing above runs on all 4050 computers and the 405x Web Browser Emulator, a version for the 405x Emulator to time the calculation, and a version for the 4052 and 4054 computers with @jdreesen's 4052 Multifunction ROM Pack which includes the TransEra Real Time Clock for timing the calculation.
I also edited the BBC_PI program to add the ability to select how many decimals to calculate. However - you will see that the BBC_PI algorithm prints pairs of digits on each pass through the FOR C% = M% TO 14 STEP -7 loop. The first value calculated and printed is 3.1 - so this means this program will always print an odd number of decimal places. My program attempts to report how many digits will be printed but is currently off a couple of digits - so my INPUT message says "approximately".
Here is the output of the BBC_PI program (with my INPUT and PRINT formatting mods) for a small number of digits:
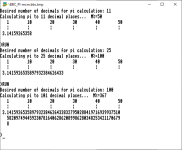
and my program results on the 405x Emulator:
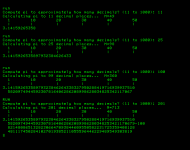
and here is a photo of my 4052 calculating Pi to 999 places with the TransEra RTC timer - 8521 seconds (2 hours 17 minutes)!
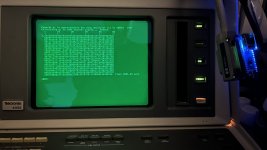
Enjoy!
Tektronix 4050 BASIC was introduced in 1975 with their 4051 vector graphics microcomputer. The 4051 and the second generation 4052 and 4054 targeted commercial, scientific and military applications and only supported double-precision floating point numeric variables. Most of the other vintage computers BASIC included integer variable support, and the PI calculation programs I found made heavy use of integer variables.
Tektronix 4050 BASIC stores floating point variables in 8-bytes, so this significantly limits the number of numeric variables in a program. I have written programs that use character strings to emulate integer variables - but this is significantly slower due to the number of BASIC commands involved. PI calculations require significant size numeric arrays.
One of the shortest and fastest BASIC programs I found for calculating PI was the one written in BBC BASIC: https://rosettacode.org/wiki/Pi#BBC_BASIC
I was not familiar with that BASIC, but I had tried porting several other programs from that webpage - and new that Tektronix 4050 computers would be performance disadvantaged, so I decided to tackle porting the BBC BASIC program to Tektronix BASIC. Here is the BBC BASIC program listing:
Code:
WIDTH 80
M% = (HIMEM-END-1000) / 4
DIM B%(M%)
FOR I% = 0 TO M% : B%(I%) = 20 : NEXT
E% = 0
L% = 2
FOR C% = M% TO 14 STEP -7
D% = 0
A% = C%*2-1
FOR P% = C% TO 1 STEP -1
D% = D%*P% + B%(P%)*&64
B%(P%) = D% MOD A%
D% DIV= A%
A% -= 2
NEXT
CASE TRUE OF
WHEN D% = 99: E% = E% * 100 + D% : L% += 2
WHEN C% = M%: PRINT ;(D% DIV 100) / 10; : E% = D% MOD 100
OTHERWISE:
PRINT RIGHT$(STRING$(L%,"0") + STR$(E% + D% DIV 100),L%);
E% = D% MOD 100 : L% = 2
ENDCASE
NEXT
This program is very short - and shows that BBC BASIC supports structured BASIC. I found a BBC BASIC for SDL 2.0 for windows here: https://www.bbcbasic.co.uk/bbcbasic.html
and found it easy to use and very quick - as it is compiled to run directly on windows.
I pasted the BBC Pi program into BBC BASIC window (I selected the Richard Russell SDLIDE version) and pressed the F9 function key and a console window popped up and began displaying the results of the Pi calculation and kept scrolling off the screen! I closed the console window and looked at the program and found one array B%() that was initialized to M%=(HIMEM)-END-1000). I tried replacing M% in the DIM B% statement with 50, pressed F9 and got an error message. I then added REM in front of the M%= calculation and added a M%=50, pressed f9 and the console window printed Pi to 11 decimal places! I then typed in the console window: PRINT HIMEM and got 192814076 (192MB)! At that point I experimented with changing the value of M% and developed an algorithm to predict the number of decimal places based on the value of M%.
I also began creating my port of the program in Tektronix 4050 BASIC using the latest experimental version of the Tek 405x (actually 4051) Web Browser Emulator:
https://forum.vcfed.org/index.php?threads/tek-405x-web-browser-emulator.62548/post-1357155
For developing my BASIC programs - I edit the mc6800.js file and replacing the 368 with 3.68 which increases the speed of the emulated Motorola 6800 CPU to about 100MHz for about 100x performance speed up of the 405x Emulator compared to the Tektronix 4051 and almost 10x speedup of the Emulator compared to my 4051 and 4052!
After hours of work - I have created this BASIC program that runs on the 405x Emulator in 32KB of RAM (and therefore should run on a 4051) and also tested it with my 4052. Since my program uses simpler BASIC calls to emulate the MOD, DIV, and print formatting of the BBC_PI program - I believe it will also be easier to port to other vintage computer BASIC dialects.
Code:
1 REM Ported from BBC BASIC (https://rosettacode.org/wiki/Pi#BBC_BASIC)
2 REM to Tektronix 4050 BASIC - mcm Jan 25, 2024
3 REM added INPUT #decimals for pi
4 INIT
100 PRI "Compute pi to approximately how many decimals? (11 to 1000): ";
110 INPUT D1
120 IF D1<11 OR D1>1000 THEN 100
130 D1=D1-1
140 M=INT((D1/2+2)/7*49)
150 D2=INT((M-13)/7)*2+1
160 PRINT "Calculating pi to ";D2;" decimal places... M=";M;" ";
170 PRINT " 1 10 20 30 40 50"
180 PRINT " | | | | | |";
190 REM NO timer
200 REM INPUT @2:A$
210 REM GOSUB 680
220 REM T=S
230 DIM B(M+1),B$(10),P$(10),Q$(10),R$(10)
240 REM use Tek 4050 array math to init B(M+1) length to store 'B(0)'
250 B=20
260 E=0
270 L=2
280 F=0
290 FOR C=M TO 14 STEP -7
300 D=0
310 A=INT(C*2-1)
320 FOR P=C TO 1 STEP -1
330 D=INT(D*P+B(P+1)*100)
340 B(P+1)=D-A*INT(D/A)
350 D=INT(D/A)
360 A=A-2
370 NEXT P
380 IF D<>99 THEN 410
390 E=E*100+D
400 L=L+2
410 IF C<>M THEN 450
420 PRINT "";INT(D/100)/10;
430 E=D-100*INT(D/100)
440 GO TO 660
450 F=F+1
460 Q=E+INT(D/100)
470 P$=STR(Q)
480 Q1=LEN(P$)-1
490 Q$=SEG(P$,2,Q1)
500 Q$="00"&Q$
510 Q1=LEN(Q$)
520 Q$=SEG(Q$,Q1-1,2)
530 IF F-25*INT(F/25)<>0 THEN 630
540 R$=SEG(Q$,1,1)
550 PRINT R$;
560 IF F-50*INT(F/50)<>0 THEN 590
570 PRINT "-";F*2
580 GO TO 600
590 PRINT ""
600 R$=SEG(Q$,2,1)
610 PRINT " ";R$;
620 GO TO 640
630 PRINT Q$;
640 E=D-100*INT(D/100)
650 L=2
660 NEXT C
670 REM no timer
680 PRINT
690 END
I have inserted blank lines in the listing for better readability. I have created several versions of this program: the listing above runs on all 4050 computers and the 405x Web Browser Emulator, a version for the 405x Emulator to time the calculation, and a version for the 4052 and 4054 computers with @jdreesen's 4052 Multifunction ROM Pack which includes the TransEra Real Time Clock for timing the calculation.
I also edited the BBC_PI program to add the ability to select how many decimals to calculate. However - you will see that the BBC_PI algorithm prints pairs of digits on each pass through the FOR C% = M% TO 14 STEP -7 loop. The first value calculated and printed is 3.1 - so this means this program will always print an odd number of decimal places. My program attempts to report how many digits will be printed but is currently off a couple of digits - so my INPUT message says "approximately".
Here is the output of the BBC_PI program (with my INPUT and PRINT formatting mods) for a small number of digits:
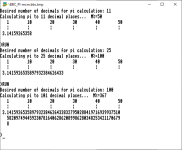
and my program results on the 405x Emulator:
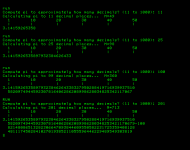
and here is a photo of my 4052 calculating Pi to 999 places with the TransEra RTC timer - 8521 seconds (2 hours 17 minutes)!
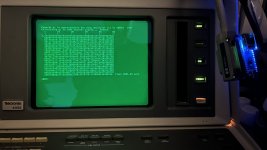
Enjoy!